在 Laravel 中使用 URI 类
Laravel 11.35 引入了 PHP League 的 URI 库驱动的 Uri 类。Uri 类使得在 Laravel 中操作 URI 更为容易,同时也为命名路由周边引入了一些便利。
基础操作
Uri 类的核心是创建和操作 URI 字符串,包括查询参数、URI 片段(fragment)和路径:
use Illuminate\Support\Uri;
$uri = Uri::of('https://laravel-news.com')
->withPath('links')
->withQuery(['page' => 2])
->withFragment('new');
(string) $url; // https://laravel-news.com/links?page=2#new
$uri->path(); // links
$uri->scheme(); // https
$uri->port(); // null
$uri->host(); // laravel-news.com
同时,也请注意获取 URI 值和解码 URI 值之间的差异:
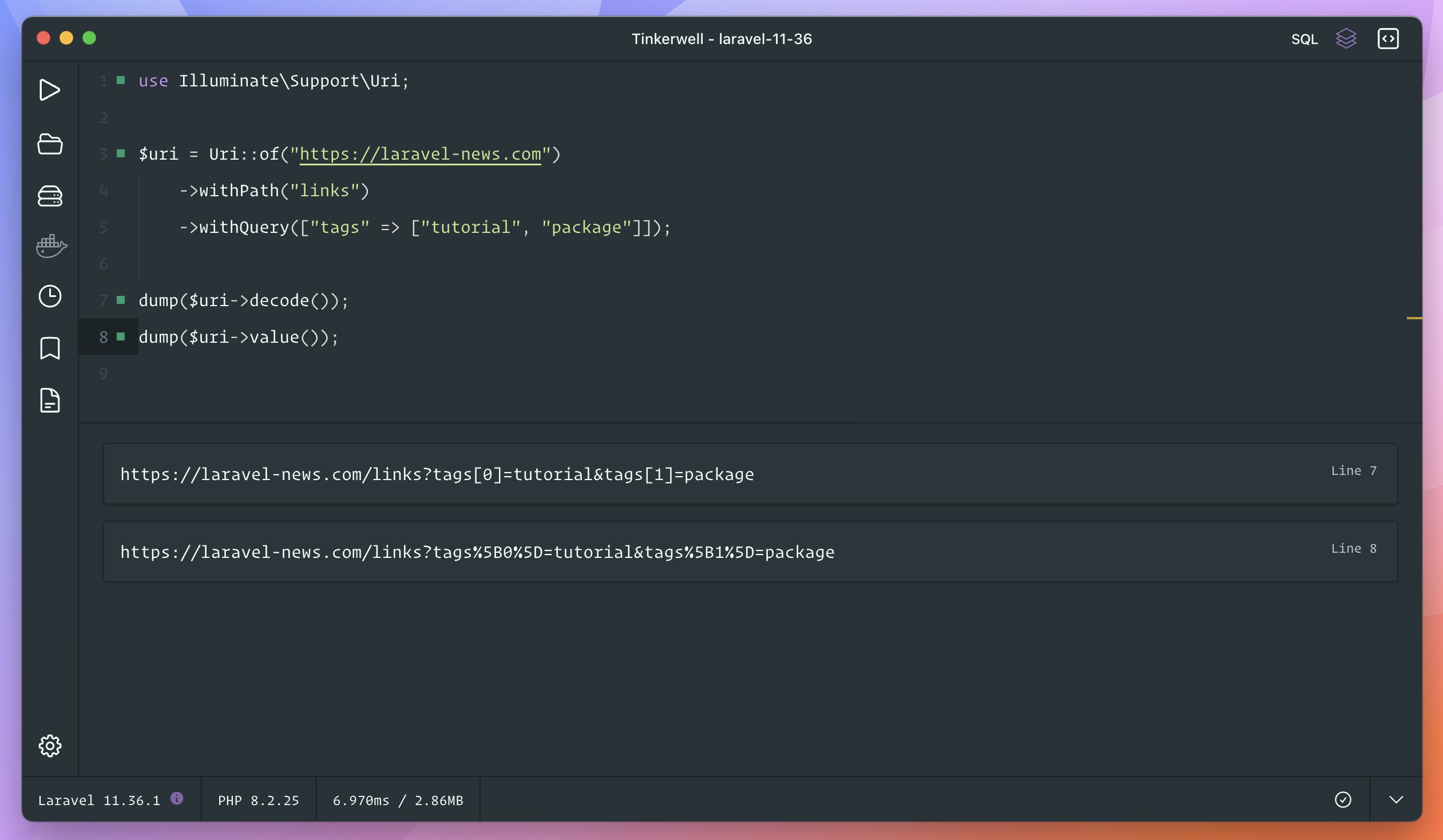
查询断言和操作
在 Laravel 中使用 UriQueryString
使得断言及操作 URI 查询参数变得更为容易。该类使用了支持性的 trait InteractsWithData
,提供了许多有用的方法用以断言查询字符串。
use Illuminate\Support\Uri;
$uri = Uri::of("https://laravel-news.com")
->withPath("links")
->withQuery(["page" => 2, 'name' => ''])
->withFragment("new");
$uri->query()->all(); // ["page" => "2"]
$uri->query()->hasAny("page", "limit"); // true
$uri->query()->has("name"); // true
$uri->query()->has('limit'); // false
$uri->query()->missing('limit'); // true
$uri->query()->filled('page'); // true
$uri->query()->filled("name"); // false
$uri->query()->isNotFilled("name"); // true
$uri->query()->isNotFilled("page"); // false
$uri->query()->string("page", "1"); // Stringable{ value: 2 }
$uri->query()->integer("limit", 10); // 10
了解 InteractisWithData
为 UriQueryString
实例提供的所有有用方法,以断言和操纵查询数据。
从命名路由、路径和当前请求中获取 Uri 实例
Uri
类还可以从应用中的命名路由、相对 URL,甚至从当前 Request
实例创建 Uri:
// Using a named route
(string) Uri::route("dashboard"); // http://laravel.test/dashboard
// Using a root-relative URL
(string) Uri::to("/dashboard"); // http://laravel.test/dashboard
// From the current request
function (Request $request) {
(string) $request->uri(); // http://laravel.test/dashboard
}
从 Laravel 11.36 开始,默认情况下 Laravel 应用中的 Uri
类已经别名的,这意味着你可以在不引入 Illuminate\Support\Uri
命名空间的情况下使用它。